Addressing Rust Security Vulnerabilities: Best Practices for Fortifying Your Code
Best practices for addressing Rust security vulnerabilities. Rust-lang vulnerability examples and remediation tips.
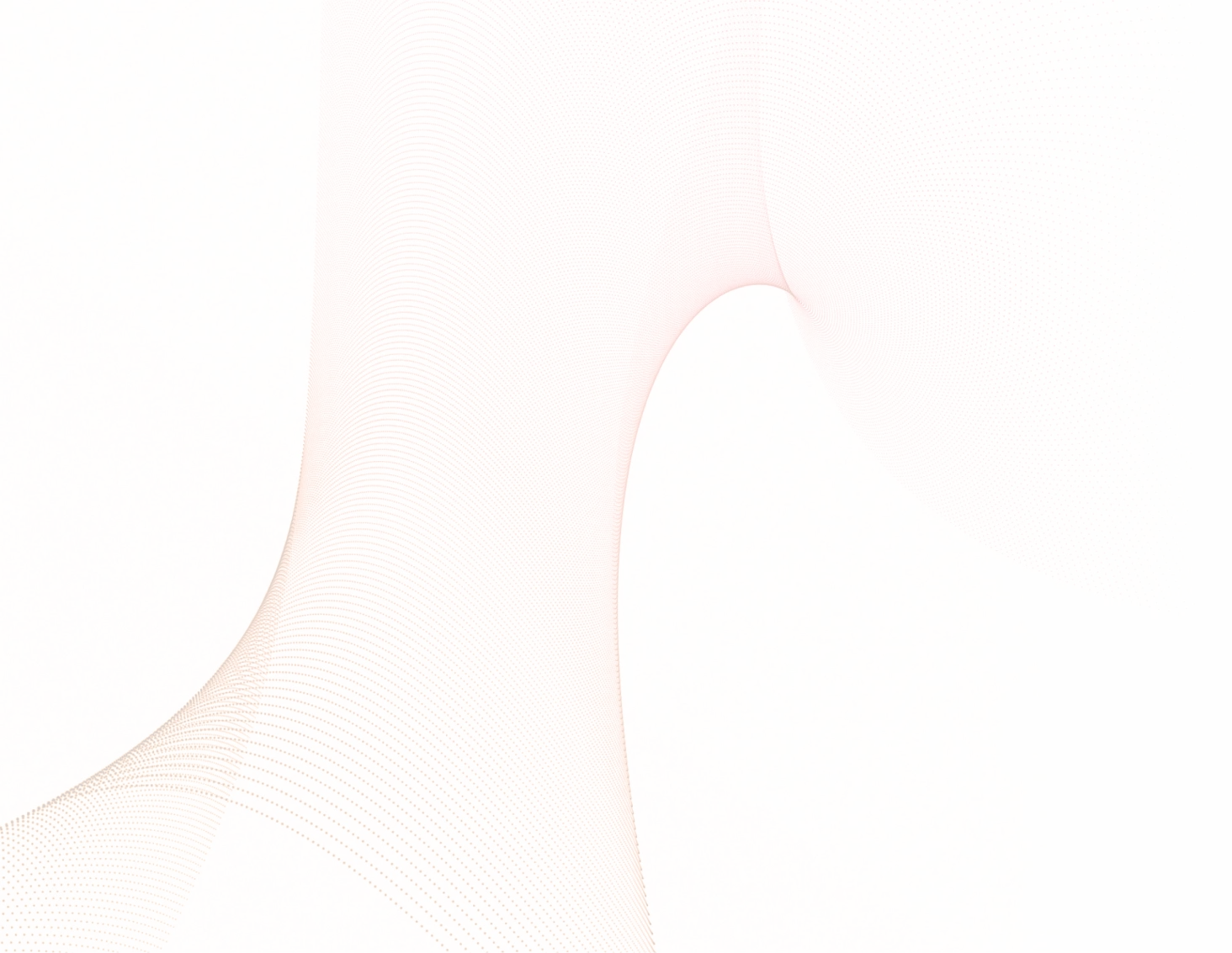

Rust has quickly gained a reputation as a systems programming language that prioritizes safety and performance. With its unique ownership model and focus on memory safety, Rust is often lauded for its ability to prevent entire classes of vulnerabilities, such as null pointer dereferencing and buffer overflows.
However, no language is entirely immune to security issues. This guide explores key security considerations in Rust, highlights specific vulnerabilities, and provides best practices to ensure your Rust applications are as secure as possible.
Examples of Common Rust Security Vulnerabilities
1. Memory Safety Issues in Unsafe Code
Rust’s most celebrated feature is its memory safety guarantees, but these guarantees are only valid in safe Rust. The use of unsafe blocks allows developers to bypass some of Rust’s safety checks, potentially introducing vulnerabilities like use-after-free, null pointer dereferencing, or buffer overflows.
Here are some examples of unsafe code commonly executed in Rust:
unsafe {
let x = *ptr; // Dereferencing a raw pointer
}
In this example, if ptr is not correctly initialized or validated, it can lead to undefined behavior, opening the door to memory corruption and potential exploitation.
Solution:
- Minimize the use of unsafe code and confine it to well-reviewed and tested modules.
- Apply additional checks and safeguards when unsafe is necessary, such as validating pointers and ensuring proper memory allocation.
2. Integer Overflow and Underflow
While Rust’s default integer operations are safe and will panic on overflow in debug mode, in release mode, this behavior changes, potentially leading to silent overflows that attackers can exploit.
CVE Reference: CVE-2018-1000810 is an example of an integer overflow vulnerability that affected the Rust standard library, demonstrating that even Rust’s core components can be susceptible.
Solution:
- Use Rust’s checked arithmetic methods, such as checked_add, checked_sub, and checked_mul, to explicitly handle potential overflows.
- Enable overflow checks in release builds using the overflow-checks = true option in your Cargo.toml file.
3. Dependency Management and Supply Chain Attacks
Like many modern languages, Rust projects often rely on a wide array of third-party crates (libraries). However, poorly maintained or malicious crates can introduce a Rust security vulnerability.
Example: A compromised or outdated crate could introduce a critical vulnerability in your Rust application, potentially leading to data breaches or system compromise.
Solution:
- Regularly audit your dependencies using tools from Kodem Security to check for known vulnerabilities.
- Leverage Kodem’s solutions to automatically monitor and update dependencies, mitigating supply chain risks.
4. Data Races in Concurrent Programming
Rust’s ownership system makes it challenging to have data races in safe Rust, but they can still occur in unsafe blocks or when using certain concurrency primitives incorrectly.
Example: A poorly implemented concurrency pattern using raw pointers or unsafe code could lead to race conditions, where multiple threads concurrently access and modify shared data.
Solution:
- Leverage Rust’s concurrency primitives, such as Arc (Atomic Reference Counting) and Mutex, to ensure safe access to shared data.
- Avoid unsafe code in concurrent contexts unless absolutely necessary and ensure thorough testing and review.
Real-World Case Study: Rust Security Vulnerabilities
Case Study: CVE-2020-36317 in the Serde Crate
A critical vulnerability was discovered in the popular serde crate, which is widely used for serializing and deserializing data in Rust applications. The vulnerability allowed for the potential execution of arbitrary code during deserialization under certain conditions.
Attack Scenario: An attacker crafts a malicious data payload that, when deserialized by the vulnerable serde version, triggers code execution, compromising the integrity and security of the application.
Mitigation Strategies:
- Regularly update your crates to the latest secure versions using cargo update.
- Audit your dependencies with tools provided by Kodem Security to catch known vulnerabilities in your Rust projects.
Best Practices for Secure Rust Programming
Embrace Rust’s Safety Features
Rust’s design prioritizes safety, so take full advantage of its features like ownership, borrowing, and lifetimes. Avoid using unsafe code unless absolutely necessary, and when you do, document and test it rigorously.
Use Type Safety to Prevent Bugs
Rust’s strong type system helps catch many potential bugs at compile time. Use it to enforce invariants and prevent invalid states that could lead to security issues.
Monitor and Audit Dependencies
Given the potential for supply chain attacks, regularly audit and update your dependencies. Tools from Kodem Security, (Runtime SCA) are invaluable for maintaining the security of your Rust projects.
Write Secure Concurrency Code
Rust’s concurrency model is powerful but requires careful handling to avoid data races and other concurrency issues. Stick to safe Rust concurrency primitives and avoid unsafe in multithreaded contexts.
Static Analysis and Continuous Monitoring
Incorporate static analysis tools like Clippy into your development process to catch potential issues early. Additionally, employ runtime monitoring and logging to detect and respond to anomalies in your Rust applications.
Conclusion
Rust is a robust and secure programming language, but like any tool, its security depends on how it’s used. By understanding the unique security challenges that Rust presents and following best practices, you can build applications that are not only high-performance but also resilient against attacks. Regular auditing, cautious use of unsafe code, and a proactive approach to dependency management are essential strategies for maintaining secure Rust applications.
At Kodem Security, we’re committed to empowering developers with the knowledge and tools they need to navigate the security landscape of Rust and other modern programming languages. With the right approach, you can leverage Rust’s strengths to build secure, efficient, and reliable software.
Feel free to send us a note with any questions >>
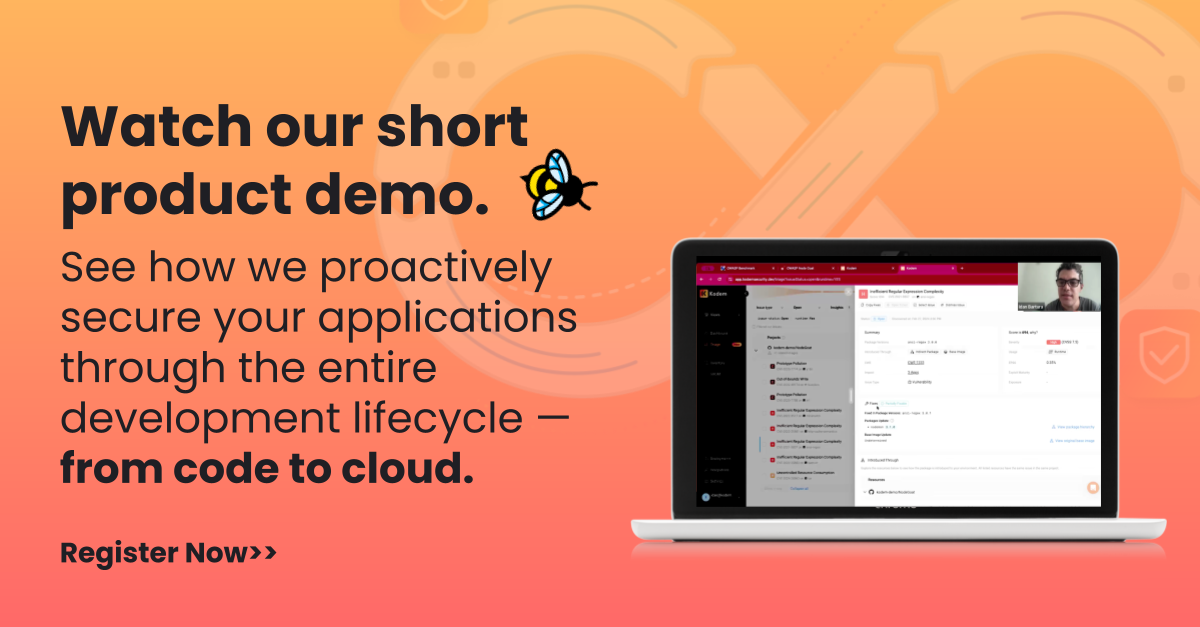
More blogs
A Primer on Runtime Intelligence
See how Kodem's cutting-edge sensor technology revolutionizes application monitoring at the kernel level.
Platform Overview Video
Watch our short platform overview video to see how Kodem discovers real security risks in your code at runtime.